Code sign using PowerShell
Code signing is important before releasing our software to the client. In the previous post, we use a PowerShell script to publish our code. After publishing the code, we need to sign the code before release. We must have code cert for signing. Install the code sign in your machine. Certificate installs process is simple using certificate import wizard.
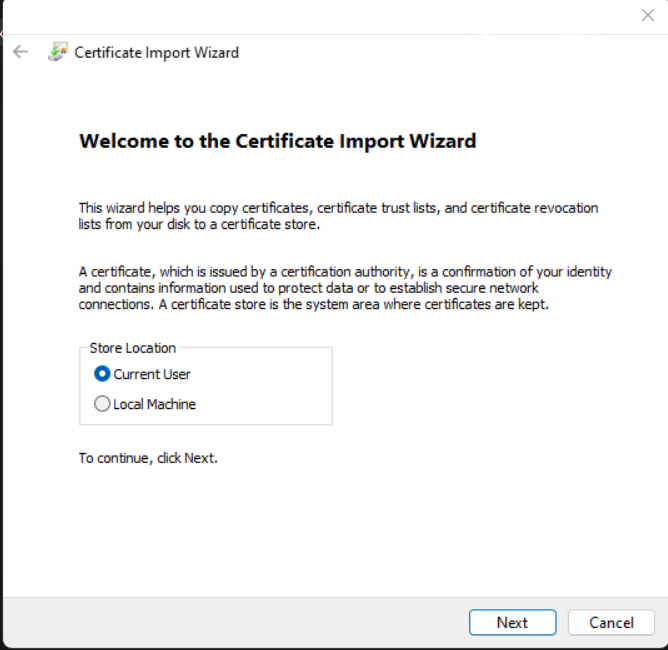
Windows Certificate installation wizard.
Once the certificate is install, we can procced to code signing process.
cd src
$paths = Get-ChildItem -include *.csproj -Recurse
foreach($pathobject in $paths)
{
cd $pathobject.directory.fullName
dotnet publish -o ..\..\__DEPLOY__\Program
}
cd ..\..
This part of the code from previous post this script publish all the project in the solution.
$cert = Get-ChildItem -Path Cert:\CurrentUser\My -CodeSigningCert
Get-ChildItem -recurse -path '__DEPLOY__\' -Include *.exe, *.dll | ForEach-Object {
$signingParameters = @{
FilePath = $_.FullName
Certificate = $cert
HashAlgorithm = 'SHA256'
TimestampServer = 'http://timestamp.digicert.com'
}
Set-AuthenticodeSignature @signingParameters
}
The first line gets the signing cert path.
Next line, navigate to the code that needed to sign, in this example path is ‘__DEPLOY__’, sign will only the files with exe and dll extension. Subsequent lines shows different params for signing. You can find more about the code signing params from PowerShell documentation.
If you want to exclude any folder and files from signing, you can define like this
[string[]]$Exclude = @('ExcudeFolderName')
Get-ChildItem -recurse -path '__DEPLOY__\Program\' -Include *.exe, *.dll | Where-Object { $_.DirectoryName -notmatch $Exclude } | ForEach-Object
cd src
$paths = Get-ChildItem -include *.csproj -Recurse
foreach($pathobject in $paths)
{
cd $pathobject.directory.fullName
dotnet publish -o ..\..\__DEPLOY__\Program
}
cd ..\..
[string[]]$Exclude = @('ExcudeFolderName')
$cert = Get-ChildItem -Path Cert:\CurrentUser\My -CodeSigningCert
Get-ChildItem -recurse -path '__DEPLOY__\Program\' -Include *.exe, *.dll | Where-Object { $_.DirectoryName -notmatch $Exclude } | ForEach-Object {
$signingParameters = @{
FilePath = $_.FullName
Certificate = $cert
HashAlgorithm = 'SHA256'
TimestampServer = 'http://timestamp.digicert.com'
}
Set-AuthenticodeSignature @signingParameters
}
Above snippet is the entire script.
Happy coding 🙂